By IDM Grad Student Amy Hu
A light sensing panel inside the lining of a jacket so that when jacket is opened, LEDs turn on to bring attention to the objects being displayed.
Materials |
• 1 LilyPad • 1 MicroUSB Cable • 3 Adafruit LED Sequins • 1 Photoresistor • 1 10K Resistor • 2 Coin Battery Holder • 2 CR2032 Coin Battery • Needle & Conductive Thread • Fabric • Alligator Clips |
Set-Up Before sewing material, test the circuit elements to make sure that they are working properly. Right click and open images in another tab to see them in a larger format. |
1. Use alligator clips to connect circuit elements between a breadboard to the LilyPad. Below is the circuit we would be testing, it uses a voltage divider to read the analog signal from the photoresistor.
On a LilyPad the analog pins have an “A” suffix and 5 digital pins. The power is the “+” and ground is “-“. 2. Open the Arduino IDE and paste the following code into the window. Connect the LilyPad to your computer with the MicroUSB cable. Make sure under the “Tools” tab, the board is “LilyPad Arduino USB” and the port with the LilyPad is selected. Connections Code ![]() ![]() |
Prototype 1 This was my first attempt of making the system, where I made the system display Pokemon Gym Badges. |
![]() ![]() ![]() Lessons Learned: For Prototype 2: |
Prototype 2 Using what I learned in my previous prototype I made a different iteration that would be run using coin cell batteries. |
![]() ![]() The above traces are first made with pencil and then filled in with conductive thread. With this layout, it’s a lot easier to see where parts are connected with one another. For the legs of the photoresistor and resistor, there is normal thread used to hold them more securely. ![]() ![]() The cell holder is connected to the “+” and “-” terminals of the LilyPad which will power the system. There is a 3.7V power requirement from the LilyPad so another coin cell battery was added in series. Note: Delay Function The “Blink” Example in the Arduino IDE calls delay() which pauses the program. With the program paused, it can cause any sensor reading for values in the loop function to be missed or skipped.Instead we’re using this work around: LEDms = millis(); With this code every half a second, it will check if the delay has passed. If so, we’d change the previous time to be the current time and write what we want to change in the if statement. For our case, we would be checking if the photoresistor is covered or not at a given time. |
Codeint photor = A2, led1 = A3, led2 = A4, led3 = A5; |
Demo |
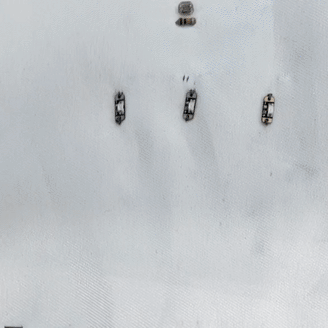
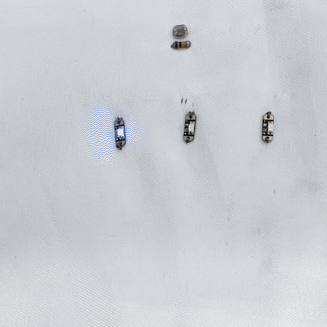

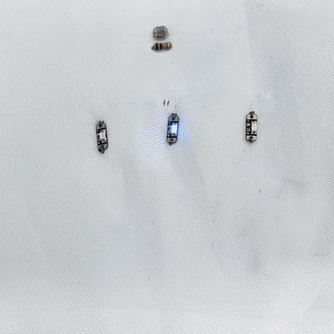